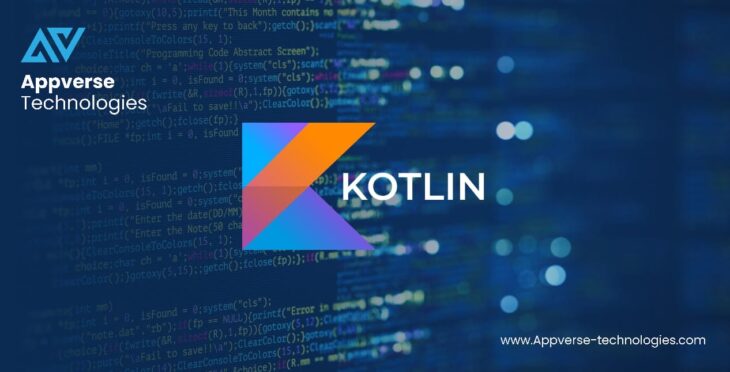
Introduction:
Kotlin is a modern programming language that is statically types language. It runs on Java Virtual Machine (JVM). It can be used for creating android applications, server-side applications and much more.
Origin and development:
- 2010: Kotlin was developed by JetBrains, a company that is famous for developing Integrated Development Environments IDEs like IntelliJ IDEA. The main goal behind developing this language was to overcome the shortcomings of Java language. But it was fully interoperable with java.
- 2011: JetBrains announced first project of Koltin publicly. The name of this language was based on an island named as Kotlin that is situated near St. Petersburg, Russia. It is the place where the JetBrains team is based.
- 2012: The first review version of Koltin language was released under the license of Apache 2. The first commitment was to release as open-source language.
Milestones:
- 2015: The first official release version Kotlin 1.0 was released after many review versions since 2012. More wide concepts were adopted in this version like stability and backward compatibility.
- 2017: In this year Kotlin earned a worthy mile stone as the Google announced its first class support for the Kotlin programming language at Google I/O conference. Also, Kotlin gained its dramatically adoption in Android Development.
- 2018: Coroutines, an asynchronous programming feature that made writing non blocking code easier and simplified concurrency, were introduced in Kotlin 1.3.
- 2019: Google declared Kotlin as preferred language for Android Development because it enhanced its position in Android ecosystem.
- 2020: Newer version Kotlin 1.4 was released with enhanced capabilities like scalability, better performance and development experience. This version includes better IDE support, an inference algorithm and enhanced multiplatform capabilities.
- 2021: Kotlin 1.5 was released with features like JVM records, sealed interfaces and support for JVM IR (Intermediate Representation). Which provided foundation for future enhancements.
- 2022: Kotlin 1.6 was released to make Kotlin language more refined. It focused more on improving the Kotlin multiplatform project, which allows for code sharing between different platforms.
Current Status:
As of 2024, Kotlin continues to evolve with regular updates that enhance its features and functionality. It is being widely used for different domains line android development, web development, server-side development etc. its powerful community makes it sure that the code
will be relevant for modern software development.
Key features of Koltin Programming Language
Kotlin is modern and statically types language. Its main features are as follows:
- Interoperability with java:
a. Seamless integration: Kotlin is fully interoperable with java, it means that java code can be called form Kotlin and vice versa. It makes it easier to move gradually form java to Kotlin and use existing libraries of java. Also, it is easy to convert code of Kotlin code into java code. When two languages coexist, productivity of developer’s level increases. Programmers also feel it easy to work with existing android projects in java using Kotlin language as conversion is easier and less risky in Kotlin. - Concise syntax:
a. Reduced Boilerplate: Kotlin’s syntax is more concise and expressive than java. This makes the code more less and makes code base read easier and maintainable.
b. Concise code: Although, Kotlin is fully compatible with java but the code written in Kotlin is comparatively less than java. According to a rough estimation, code in Kotlin is about 40% less than java. Less code is always loved and appreciated by
developers for developing and reading the code. - Extension function:
a. Adding functions to existing classes: When a function is added to an existing class, it is called Extension function. Kotlin allows the programmer to add extension functions to existing classes without modifying their existing code. It makes existing libraries more useful. Also makes the code more readable for developers. - Coroutines for Asynchronous Programming
a. Simplified asynchronous code: Kotlin provides coroutines, which simplify asynchronous programming by allowing you to write sequential code that is non blocking and efficient.
b. Coroutines in Kotlin: Coroutines in Kotlin is like threads used for cooperative multitasking in other languages. It also allows switching from one execution context to another execution context. Coroutines also used for suspending functions to switch from suspension point to any other point in code.
c. Suspension function: suspension functions are the functions that have one or more suspension. Suspension is the point where the execution of the code is paused to be resumed after some moments. - Type interference
a. Less verbose code: Kotlin supports type interference, it means that the compiler automatically deduces the data types of variables, reducing explicit type declarations.
b. Late initialization: But if you want to initialize the variable late, then you have to
specify the data type. At this point type inference concept do not work. - Smart casts:
a. Automatic type casting: Kotlin automatically performs type casting functions when the need arises based on the conditions and checks. It performs all the coded function for a specific type and also causes the efficiency of the code. It also speeds up the performance of the application. - Null safety:
a. Eliminating NullpointerExceptions: in Kotlin, variables and objects are non nullable by default unless explicitly declared as nullable. In other programming languages about 70% crashes cause the flopping of the application. But Kotlin’ feature of null safety avoid any nulls in programming until assigned implicitly. - Data classes:
a. Simplified model classes: Data classes are the common features that are widely used in development specifically in android development. In java, there is no any way to make difference between data classes except their structure and pattern. But there is no any case in Kotlin language. Data classes in Kotlin provides the facility to use less code for data holding and automatically generates the boilerplate functions like ‘equals()’, ‘hashCode()’ , ‘toString()’ etc. - Standard library:
a. Rich API: Kotlin provides a rich set of APIs, string manipulations, I/O operations and much more for providing a rich toolkit for developers. The rich library of Kotlin provides a treasure trove for developers who take benefits from it and make their apps more efficient. - Multiplatform development:
a. Code sharing across platform: Kotlin enables the reuse of code by sharing code across different platforms like Android, iOS, Native etc. code written for one platform can be executed on different platforms. It also appreciates the reuse of the code.
Conclusion:
These core features make Kotlin a powerful language specially for android development, backend development and cross-platform mobile application development. It is especially better tool for android developers. With its feature like standard library makes the code more reusable and readable for developers.
Getting starts with Kotlin
Install Kotlin:
There are many IDEs for writing programs in Kotlin but IntelliJ IDEA is good choice for new and experienced programmers. Here two options are going to be discussed for inatalling Kotlin on your system.
Option1: Using IntelliJ IDEA
- Downloading and installing IntelliJ IDEA:
• Visit IntelliJ IDEA download page and download suitable edition whether community or Ultimate edition.
• Install IntelliJ IDEA according to the instruction by your operating system. - Create new project:
1. Open IntelliJ IDEA and choose new project form the welcome screen.
2. Another option is to go to File->New->New Project.
3. Name the project and change the location if required.
4. Select Kotlin as language form the list.
5. Choose the build system from IntelliJ. Sine it is native builder, no extra files to be downloaded. Choose Maven or Gradle if you wish to create more complicated project that requires additional settings. Select groovy or Kotlin for the build script language is Gradle.
6. From the JDK list, select the JDK which you wan to use in your project.
7. Check the boxes like Add sample code if required.
8. Click create.
Option 2 : using command line
- Install Kotlin compiler:
• Download the Kotlin compiler form the Kotlin website.
• Unzip the files and add the path of ‘bin’ folder to the ‘Path’ variable in system variable in advanced system setting in your system setting. - Write Kotlin code:
• Create new file named as ‘hello.kt” or as you wish.
• Open this file in your editor and write code as described below:
fun main() {
println(“Hello, World!”)
} - Run this code:
• Open command line or termial.
• Navigate to the desired file at the desired location and run following code: kotlinc Hello.kt -include-runtime -d Hello.jar java -jar Hello.jar
Basics of Kotlin language
Variables:
In Kotlin language the variables are declared using two ways.
- val keyword:
Using this keyword, variables are declared with no changeable value. The values of these types of variables cannot be modified during program execution. It is also called immutable. Syntax is as follows:
fun main(){
val n=10
println(“Value is:” +n)
}
Output:
Value is:10
Unlike other languages, the code statements are not ended with semicolon in Kotlin language. - var keyword:
variables declared using var keyword can be modified again in the rest of the program. Sample code is as follows:
fun main(){
var n=10
println(“previous value is:$n”)
n=12
println(“current value is:$n”)
}
Output:
previous value is:10
current value is:12
Type inference:
In Kotlin programming language, it is not necessary to specify the data type of the variable. The compiler infer the data type of the variable by its own at the time compilation and execution. It does not mean that the specifying data type is not allowed in Kotlin. It can be done
as described in following example:
fun main(){
var n = “name”
var age: Int=24
}
In the above code, the data type of first variable at the time of compile time will be automatically detected as String. And the data type of second variable is specified so the compiler does not have to detect it automatically.
Nullable variables:
By default, it is not legal in Kotlin language to remain the variable null. It helps the system to prevent from null point exception. But the variables can be initialized as null by the programmer. By default, it is not possible. For example:
fun main(){
var nonNullable: String = “Hello”
//nonNullable = null // Error: Null cannot be a value of a non-null type String
var nullable: String? = “Hello”
nullable = null // This is allowed
println(nonNullable)
}
Output:
null
Explanation:
In the above code if the line number 3 is uncommented then the compiler generates an error because it is not allowed in Kotlin. But is we do this using second method defined in line number 4 and 5.
Late initialization:
Sometimes it is not must to initialize a variable at the time of its initialization. For this purpose, a keyword of latinit is used with variable at the time of declaration. But it can be used just for var variables. As described in the following example:
lateinit var str:String
fun initString(){
str=”some string here”
}
fun printString(){
if(::str.isInitialized){
println(“your string is: $str”)
}
else{
}
}
fun main(){
println(“string is not initialized”)
printString()
}
Output:
string is not initialized
Explanation:
In the above code, a global variable that is accessible from anywhere in the whole program is declared at the beginning of the program. Two functions named as printString() and initString() are defined each for a separate purpose. In the main function only one function has been called printStirng(). If initStirng() function is called first, the string type variable contains some value but the function printStirng() is called only which means that there is no value in it. And the variable may exist without having a value.
Constants:
Now its time to discuss another important concept called constants in Kotlin. These variables are compiling time constants which means these cannot be changed at compile time once initialized. These are declared and initialized using keyword of const before var or val keywords. Look at the following example:
fun main(){
const var n= 40
n+=3 //compile time error because constant cannot be modified.
}
the above program will generate an error at the line number 3 because to change a constant variable in Kotlin is illegal. These constants are useful where the developer does not want to value to be changes by the user.
Data types:
Although there is no need to specify the data type of the data type but if one wants to specify it can be done. Different data types in Kotlin are mentioned in following table:
- Numbers:
Number data types are divided into two branches:
a. Integers:
These data types store whole numbers without floating point values. Valid data types are “Byte”, “Short”, “Int” and “Long”.
b. Floating point values:
These data types include numbers with decimal point values. Valid data types are “Float” and “Double”. - Boolean:
This data type allows a programmer to store only one value either true or false. - Characters:
This type of data type is used for storing one character at a time in single quotation mark. - String:
This data type is used for storing a series of characters in double quotation mark.
Comments:
Like other programming languages, Kotlin also provides the facility to write comments in the code for making it more readable and understandable. Comments in any programming language are non-executable statements if the code. These are only added to make the code readable for other programmers for the programmer also. Comments in Kotlin are also written like java. It means that there are two ways to write comments in Kotlin. These are described as below:
//single line comment
/*multiline
Comment*/
Functions:
Functins are very important part of any programing language. It makes the programming language more broader and able the programmer to develop the code accroding to his own choice. Lets have a look at syntax of the fucntions in Kotlin:
Syntax:
Functions in Kotlin language are defined using a keyword fun. Function name, its paramenters and return type is specified next to the keyword. Lets have a look at the following code:
//defining the fucntion
fun Sum( a:Int, b:Int) :Int{
var num:Int= a+b
return num
}
fun main(){
//calling the fucntion
var num1=Sum(4,5)
println(“Sum of 4 and 5 is:”+num1)
}
Output:
Sum of 4 and 5 is: 9
Explanation:
The execution is always starts form the main function. In the main functio a variable named num1 is initialized. At the time of its initialization the function defined above the main funciton is called. The control will go to the funciton definition to perform the desired task and return the result as the return type is specified in the funciton prototype. If no value is returned , comipler will generate an error and will demand a value to be returned. After function execution, the control will come back to the next statement and will print the output on the screen.
Function parameters:
A function may or may not contain parameters. If it contains parameters, each paramenter is defined in parenthses and data type of each parameter is specified. Default value can aslo be assigned to parameter and can be changed in the function call.
fun printString( str:String=”Guest”){
println(“Hello $str”)
}
fun main(){
printString()
printString(“ABC”)
}
Output:
Hello Guest
Hello ABC
Explanation:
A function is kotlin can be called without parameters evne if paramaneter are defined in the function prototye. This thing is elobrated in the above program. At the first function call, there is argument has been passed but the function call has been executed successfully and print the desired output on the screen. At the second function call, an argument has been passed an the output has been generated according to the argument to be passed.
Return Types:
Return typs of function is specified after the parantheses. If there is no return type then it can be ommitted in function definition.
Varage Function:
If there is no any idea about the parameters of the function then varag keyowrd is used with the funstion before the variable name. for example:
fun printAll(vararg messages: String) {
for (message in messages) {
println(message)
}
}
fun main() {
printAll(“Hello”, “World”, “Kotlin”, “Rocks”)
}
Output:
Hello
World
Kotlin
Rocks
Explanation:
This program is showing that a single keyword vararg is used for multiple arguments. It means that this keyword allows a programmer to pass a number of arguments as required. The compiler at the function call will pass all the argumnets to the function definition and in the
function definition, a loop will print all the arguments one by one on the scrreen.
Conclusion:
Functions are the most important feature of any programming language. But the concept of functions in Kotlin language is much broader than other languages. Kotlin provides a range of properties for fucntion definition, calling a function and for other operations.
Arrays:
Arrays are used when we have to declare more than one variable of the same data type. Arrays prevent the declaration and initialization of variables by using single statement. Array is declared and initialized by using following method:
var students= arrayOf(“Zainab”, “Bisma”,”Fahad”, “Nazish”)
Conditinals and loops in kotlin
Conditionals:
Conditionals are the checks that allw a programmer to execute a statement or set of statements depending upon the condition. Basic if conditional in kotlin is as follows:
fun main(){
var num=5
if(num<5)
println(“ the number is less than 5”)
} //nothing will be shown because the condition is false
To add some alternate code in cae of false condition, else keyword is used for executing alternate statement(s). For example
fun main(){
var num1= 50
var num2= 10
var res=num1%num2
if(res==0){
println(“$num1 is multiple of $num2”)
} else{
}
}
Output:
println(“$num1 is not multiple of $num2”)
50 is multiple of 10
Explanation:
In the above code, firstly an arithmetic operator called modulus operator has been performed. In the conditional if, the value of the res variable is checked. If the the condition is true, the statements under the if will be executed. Otherwise the statements under the else will be executed.
Multiple if-else statements can aslo be used but it makes the code more complex. If need arises then one can use multiple if-else can be implemented.
Kotlin language has another conditional called when. This is like switch sturcture of java. Structure of this conditional with example is as follows:
fun main(){
val month=7
when (month){
1-> println(“January”)
2-> println(“February”)
3-> println(“March”)
4-> println(“April”)
5-> println(“May”)
6-> println(“June”)
7-> println(“July”)
8-> println(“August”)
9-> println(“Spetember”)
10->println(“October”)
11-> println(“Novermber”)
12-> println(“December”)
else-> println(“Invalid number”)
}
}
Output:
July
Explanation:
In the above program, the value assigned to the month variable will be check with all the case numbers. To which case the value matches, that value will be printed on the screen. If no value matches, the else part will be executed and the statement “Invalid number” will be printed on the screen.
Conclusion:
Conditionals are very important part of any programming language. It can be said that these are one of the main parts of the programming language. Kotlin provides many options for conditional statements. A programmer can use any option according to his desire.
Loops:
Loops are the structures to execute a statement or set od statements again and again for a specified number of time or unlimites number of time. In Kotlin language there are following basic loops:
While loop:
It is the basic loop in Kotlin language. It executes statement(s) for a specified number of times. Structure of this loop with example is as follows:
fun main(){
var num=1
while(num<=5){
println(num)
num++
}
}
Output:
1
2
3
4
5
Explanation:
At the execution of the while loop, the condition will be checked. If it is true the statements under the while loop will be executed. This process will be repeated until the condition remains true. At the stage where the condition becomes false, the control comes out of the while loop and the programm is terminated because there is no any statement after the loop. If there exists some more statements, those will be executed after loop exeution.
Do-while loop:
This is second basic loop in kotlin language. Basic difference between while and do-while loop is that do-while loop its statements at least once even if the condition is false. Structure of this loop with example is as follows:
fun main(){
var num=1
do{
println(num)
num++
}while(num<=5)
}
Output:
1
2
3
4
5
Explanation:
Firstly the value of the num variable will be printed on the screen which is one in this case. After this the condition in the loop statement will be checked. If the condition is true, the loop will be executed again. But if the condition is false, the control will terminate the execution of the loop. Program execution will also terminated because there is no any statement after the loop.
For loop:
For loop is differnet from other langauges. It is only used when works with arrys in Kotlin language. Structure of this loop with example is as follows:
fun main(){
var students= arrayOf(“Zainab”, “Bisma”,”Fahad”, “Nazish”)
for( x in students){
println(x)
}
}
Output:
Zainab
Bisma
Fahad
Nazish
Explanation:
In this program, an array is created. Then for loop will be executed. Until the value of variable x becomes null, the loop will execute again and again. And when the value of x becomes null, the compiler will terminate the loop execution.
Conclusion:
Loops are also very important programming concept. in developing programs, if there is need to execute a statement(s) again and again then loops are the best option.
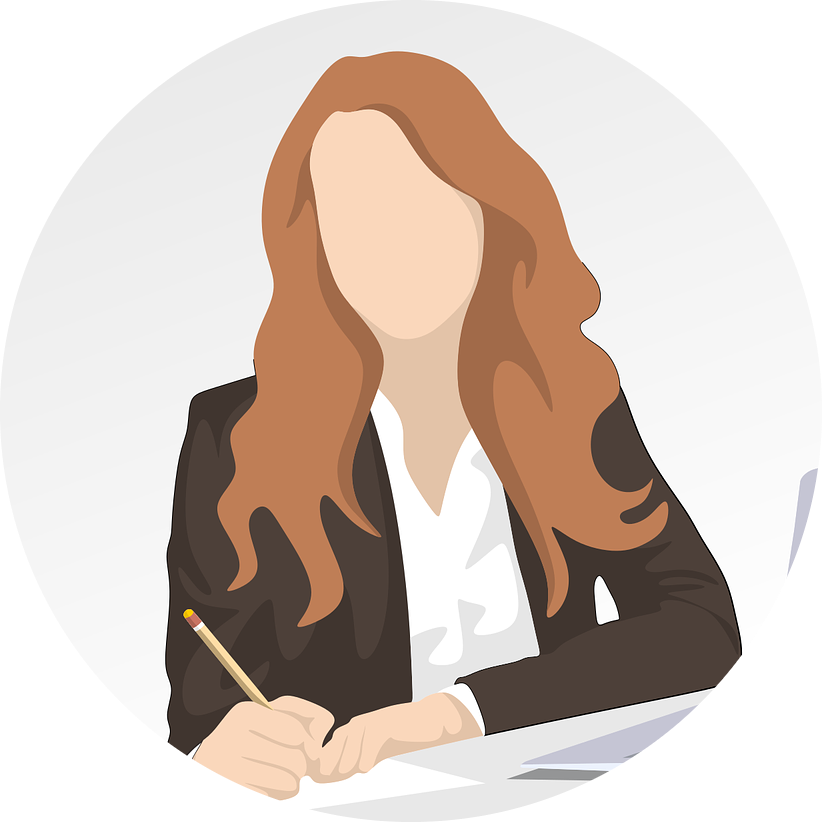
Alizay Ali is a skilled HR manager with two years of experience at AppVerse Technologies. With her strong interpersonal skills and expertise in talent acquisition, employee engagement, and HR operations, she plays a pivotal role in fostering a positive and productive work environment. She with a passion for learning and a drive to succeed, she eagerly embraces new challenges and is poised to make her mark in the ever-evolving world of technology