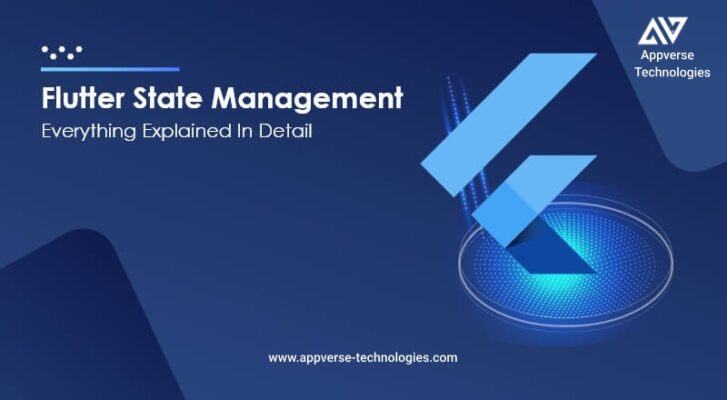
Introduction to State Management
State management is a crucial aspect of building robust and responsive applications in Flutter. It involves managing and updating the state of widgets to reflect changes in data or user interaction.
How does state management work?
Overall, state management makes the state of an app visible in the form of a data structure, improving developers’ ability to work with the app. State management libraries provide developers with the tools needed to create the data structures and change them when new actions occur.There are two accepted models for state management: Front end, or client side, and back end, or server side.In front-end state management, the user’s own app or browser maintains the program’s state, often by having certain buttons or user interface features enabled or disabled and sending the state along with the message. The user interface connection ensures the user and the application are in harmony
throughout the session.
In back-end state management, an application component uses an external data structure or database to record the final state when it’s done processing activities. Processing the next message starts with retrieving the previous state from the database. The state variable in the data structure can also synchronize the user interface — and through it the user — with the state of the session.
Why State Management Matters
Effective state management ensures that your application remains scalable, maintainable, and performs well. It helps in separating business logic from UI, making your codebase cleaner and easier to debug.
Understanding Flutter’s StatelessWidget
Flutter’s StatelessWidget is a foundational widget used for building UI components that do not require managing mutable state. Unlike Stateful Widgets, which maintain internal state and trigger UI updates, StatelessWidget is immutable and renders its UI based solely on the configuration provided during its creation.
Characteristics of StatelessWidget
Immutable Nature: Once instantiated, a StatelessWidget cannot change its internal state. It relies entirely on the data passed to it through its constructor or inherited from its ancestors. Build Method: The build() method is mandatory for StatelessWidget. It defines the UI structure of the widget based on the provided data and returns a widget tree.
Statelessness: StatelessWidget does not hold any mutable state variables or maintain any stateful data. It is typically used for static UI components that do not change based on user interactions or data updates.
Understanding Flutter’s Stateful Widget
Flutter’s Stateful Widget is a fundamental building block for managing state within Flutter applications. Unlike Stateles Widgets, which are static and do not hold any mutable state, Stateful Widgets can maintain mutable state and trigger UI updates when this state changes.
Understanding Flutter’s Stateful Widget
Flutter’s Stateful Widget is a fundamental building block for managing state within Flutter applications. Unlike Stateles Widgets, which are static and do not hold any mutable state, Stateful Widgets can maintain mutable state and trigger UI updates when this state changes.
Lifecycle of a Stateful Widget
When a Stateful Widget is first instantiated, Flutter calls its createState() method to create an associated State object. This State object persists throughout the lifetime of the widget.The State object goes through a series of lifecycle methods that Flutter calls at specific points
during the widget’s lifecycle:
initState(): Called when the State object is created. It is used for one-time initialization tasks, such as initializing state variables.
didChangeDependencies(): Called when the dependencies of the State object change. It can be used to perform additional setup that depends on the current configuration.
build(): Required method that Flutter calls to construct the UI hierarchy for the widget based on its current state. This method returns a widget tree that represents the widget’s UI.
setState(): A crucial method that triggers a rebuild of the widget when the State’s internal state changes. When called, Flutter schedules a build operation to update the UI based on the new state.
dispose(): Called when the State object is removed from the widget tree permanently. It is used to release resources or perform cleanup tasks, such as closing streams or disposing of controllers.
State Management Approaches in Flutter
setState() Method
Flutter’s setState() method is a built-in way to manage state within Stateful Widgets. It triggers a rebuild of the widget subtree when the state changes, updating the UI accordingly. This approach is straightforward and suitable for small applications or when dealing with minimal UI state changes. However, it can lead to complex and nested callback structures in larger apps, impacting maintainability.
Provider Package
Provider is a simple yet powerful state management solution in Flutter that leverages InheritedWidget for managing state. It allows for efficient data sharing between widgets without needing to rebuild the entire widget tree. Provider is ideal for managing app-wide state or sharing data across multiple widgets. Its simplicity and flexibility make it a popular choice among Flutter developers for both small and medium-sized applications.
Riverpod
Riverpod is an advanced state management library that builds upon the concepts of Provider but offers additional features such as dependency injection and a more modular architecture. It improves upon some limitations of Provider, providing better scalability and testability for larger applications. Riverpod encourages a more declarative and organized approach to state management, making it easier to manage complex app states and dependencies.
Bloc Pattern
The Bloc (Business Logic Component) pattern in Flutter is based on reactive programming principles using Streams and StreamBuilders. It separates business logic from UI components, making it suitable for managing complex state and intricate UI interactions. Bloc enhances code reusability and testability by promoting a clear separation of concerns. It’s particularly effective for apps that require extensive state management and business logic, ensuring a maintainable and scalable codebase.
Choosing the Right Approach
Selecting the appropriate state management approach in Flutter depends on factors such as app complexity, team familiarity, scalability requirements, and the specific use case. Each approach offers unique advantages and is suited for different scenarios. By understanding
their strengths and limitations, developers can make informed decisions to optimize app performance and maintainability.
Conclusion
Mastering state management in Flutter is essential for building high-quality applications that deliver a seamless user experience. By understanding the principles and selecting the right approach, developers can create maintainable, scalable, and performant Flutter applications.
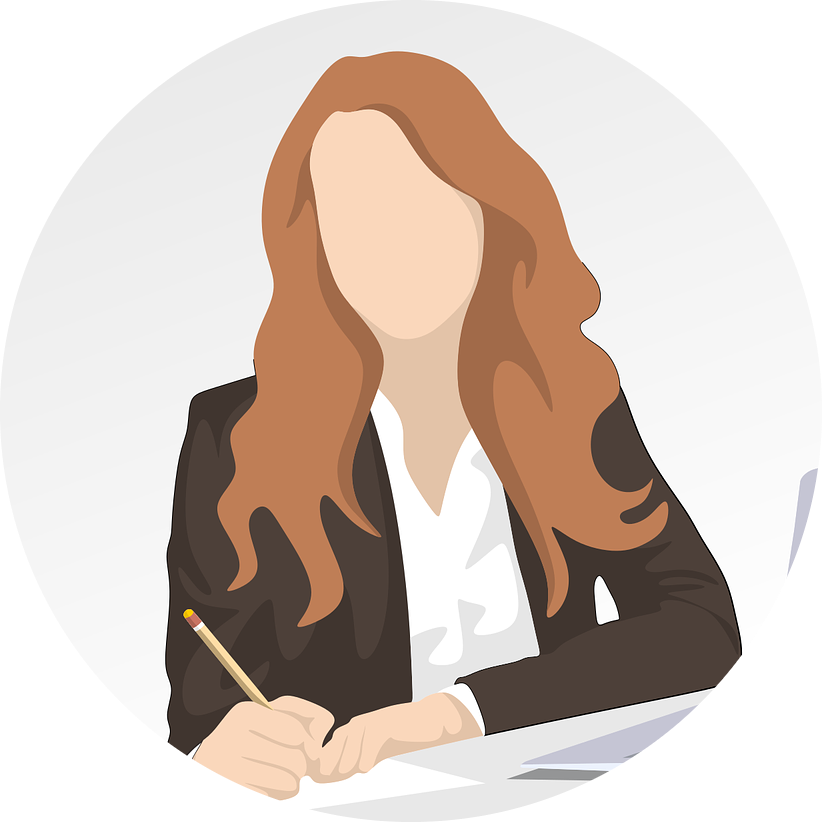
Alizay Ali is a skilled HR manager with two years of experience at AppVerse Technologies. With her strong interpersonal skills and expertise in talent acquisition, employee engagement, and HR operations, she plays a pivotal role in fostering a positive and productive work environment. She with a passion for learning and a drive to succeed, she eagerly embraces new challenges and is poised to make her mark in the ever-evolving world of technology